Getting Form Data
Collecting user-input data from Forms
Intro
Fieldwire 'Forms' is a premium module that allows users with Business and Premier subscriptions to replace paper forms with digital versions that are fast to create and distribute, easy to fill out in the field on our mobile apps, and housed permanently in a searchable Fieldwire database for improved accountability.
This guide aims to explain how to collect user-input data from forms via the API. While technically possible, it is strongly not recommended to use the API to build forms or form templates.
note: the scope of this guide is limited to getting form instances, and will not cover managing form templates via the api
Form Preparation
Due to the flexibility of the forms data model, the easiest way to gather the data within a particular form is to take a two step approach that includes:
- Triggering a full form job the /full endpoint
- Retrieving the form using the job_id returned from step 1
- If the form is not ready, you may need to poll the /full endpoint again
Example
Step 1
Call
GET /api/v3/projects/{project_id}/forms/{id}/full
Response
{
"jid": "221d6504-d50b-408f-ade3-769102920f29"
}
Step 2
Call
GET /api/v3/projects/{project_id}/forms/{id}/full?jid=221d6504-d50b-408f-ade3-769102920f29
Response
(see next section for parsing response)
Form Parsing
The response returned by the /full endpoint will contain all form data available. In order to properly understand the response, we must first outline the hierarchy of form fields:
Note: for more info about how forms work, see our help article
Form Sections
The form section represents an explicit section of the form, and may be one of 5 different types:
Depending on the form template of the form you are trying to read, you may see one or more of these sections in your json payload. Note that the order of the sections may not be sequential in your API response. In this case, you may need to review the ordinal
field to determine the section order.
As you move further down the hierarchy you will see different data structures based on the type of form sections. See below for explanations on how each are structured
List Sections
Form lists contain a series of entries that can be filled out by end users. This is represented in the API response in an array titled form_section_records
For each row in the list, you will see a corresponding form_section_record
which contains the name of the row, the order in which it appears and the items further down in the hierarchy.
Within the form_section_record
you'll see two additional arrays that describe the inputs and the values for the records
form_section_record_inputs
will contain the inputs available on the recordform_section_record_values
will contain the values entered for each input- In the
form_section_record_values
object, you'll see an arrayform_section_record_input_value
that contains the actual recorded data for each input in the section record- note that the data will be in an object called
data
and may contain null values depending on the type of the data input
- note that the data will be in an object called
- In the
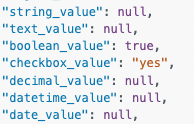
in this case, the input was a checkbox type and the user had selected "yes" on the checkbox
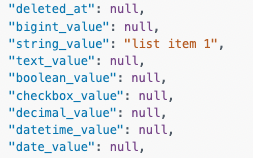
in this case, the input was a text type and the user had entered "list item 1" in the text box
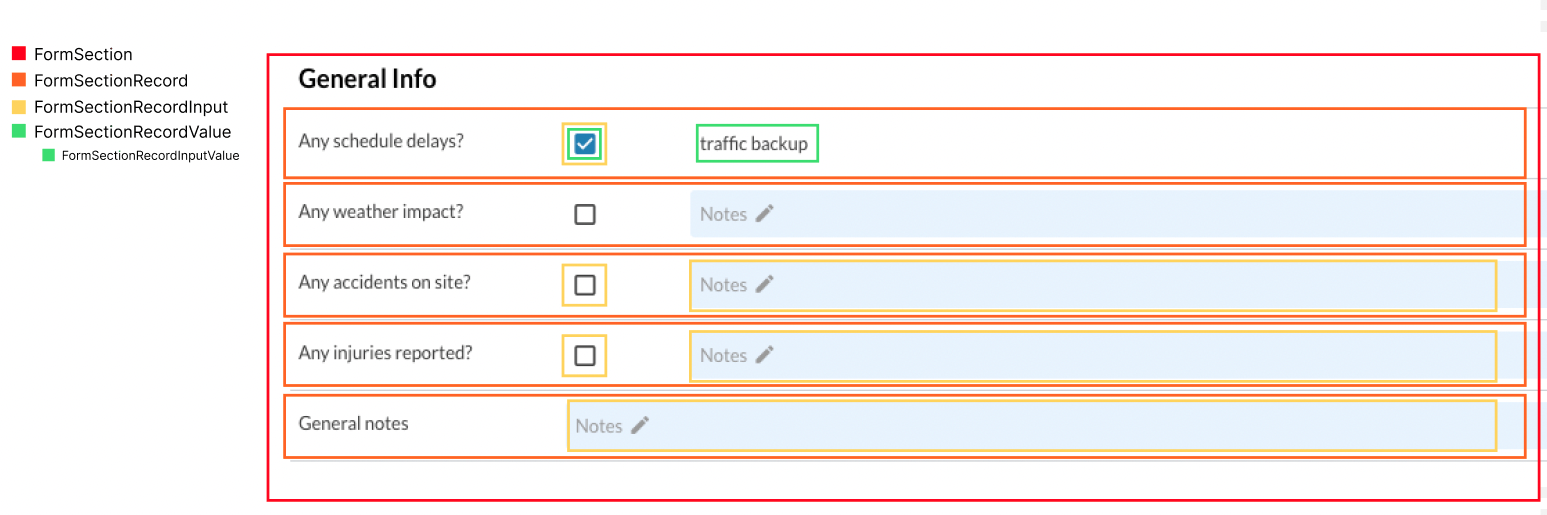
A breakdown of the various fields in a Form List section
Table sections
Form tables contain a customizable table with user defined columns and data entry types. The columns and data entry types are defined in the form template, but the form instance may have an unlimited number of rows in the table. While the API fields are similar to what you may find in list sections, the structure can be quite different.
Unlike list sections, in tables the form_section_record
represents the full table. Within that form_section_record
you will see both the form_section_record_inputs
which represent the columns, and the form_section_record_values
which represent the rows.
form_section_record_values
will contain an array with each item respresenting a single row. Within each form_section_record_value
you'll find the form_section_record_input_value
array which represents each "cell" from that row.
Note: the order of these rows and columns is not guaranteed. Use the ordinal
field to determine proper data order
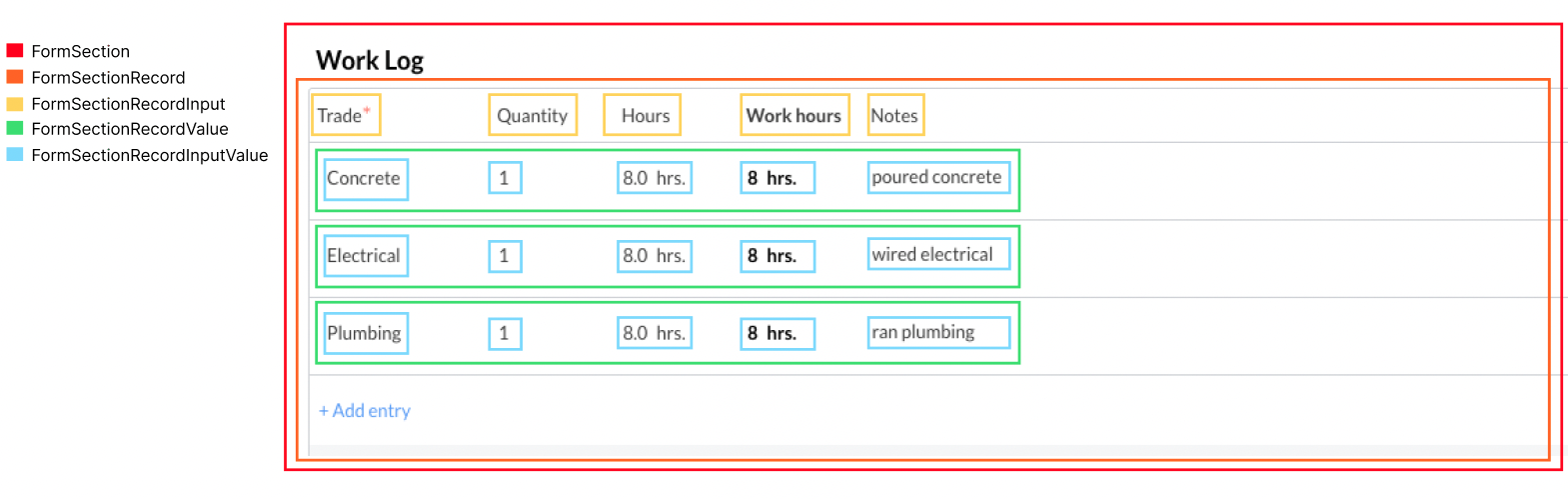
A breakdown of the various fields in a Form Table section
A note about form section IDs
If you have multiple instances of a form all created from a single template, you may want to collect data across all of these forms from a particular form section. For example, if you have the Daily Log form, you may want to collect data from all the "Work Log" sections of the form instances in your project.
Because the form section IDs are unique to the instance of the form, you cannot use that to match form sections across the different instances. Instead you can use the `form_template_section_id to do that matching as these will be preserved across all instances of the same form template
Updated about 1 year ago